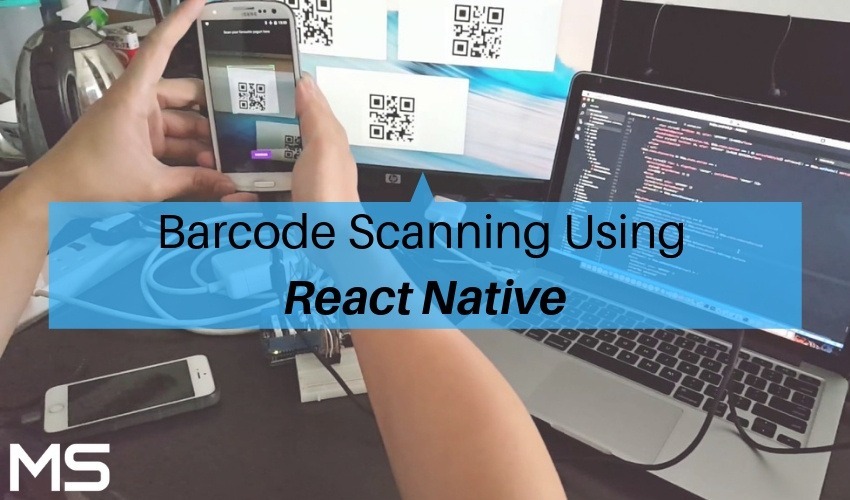
React Native Barcode Scanner comes as a great utility for scanning barcodes. While it runs fine on Android, you should use something like react-native-camera for iOS.
The postfix -Google is added since the native implementation is based on Google’s Barcode API: https://developers.google.com/vision/barcodes-overview
Table of Contents
Let’s see how it works
- How to install Barcode Scanner using React Native?
To include the latest version (1.4.0) of react-native-barcode-scanner in your project, run the following terminal commands in your React Native project root folder.
1>npm i react-native-barcode-scanner-google –save 2>react-native link react-native-barcode-scanner-google
- Benefits of using this barcode scanner
- Faster
- More accurate
- More convenient ( supports scanning in any direction)
Note that this barcode scanner doesn’t ship with a fancy overlay to display a scanning interface to the user. Instead, it’s just a fast scanner view that shows the camera stream, on top of which you can overlay your own UI.
Simple Usage
importReact, { Component } from ‘react’; import{ AppRegistry, StyleSheet, Text, View, Alert } from ‘react-native’; importBarcodeScanner from ‘react-native-barcode-scanner-google’; exportdefaultclassBarcodeAppextendsComponent { render() { return( <View style={{flex: 1}}> <BarcodeScanner style={{flex: 1}} onBarcodeRead={({data, type}) => { // handle your scanned barcodes here! // as an example, we show an alert: Alert.alert(`Barcode ‘${data}’ of type ‘${type}’ was scanned.`); }} /> </View> ); } } AppRegistry.registerComponent(‘BarcodeApp’, () =>BarcodeApp);
Advanced Usage
import React, { Component } from ‘react’; import { AppRegistry, StyleSheet, Text, View, Alert } from ‘react-native’; importBarcodeScanner, { Exception, FocusMode, BarcodeType, pauseScanner, resumeScanner } from ‘react-native-barcode-scanner-google’; export default class BarcodeApp extends Component { render() { return ( <View style={{flex: 1}}> <BarcodeScanner style={{flex: 1}} onBarcodeRead={({data, type}) => { // handle your scanned barcodes here! // as an example, we show an alert: Alert.alert(`Barcode ‘${data}’ of type ‘${type}’ was scanned.`); }} onException={exceptionKey => { // check instructions on Github for a more detailed overview of these exceptions. switch (exceptionKey) { caseException.NO_PLAY_SERVICES: // tell the user they need to update Google Play Services caseException.LOW_STORAGE: // tell the user their device doesn’t have enough storage to fit the barcode scanning magic caseException.NOT_OPERATIONAL: // Google’s barcode magic is being downloaded but is not yet operational. default: break; } }} focusMode={FocusMode.AUTO /* could also be TAP or FIXED */} barcodeType={BarcodeType.CODE_128 | BarcodeType.EAN_13 | BarcodeType.EAN_8 /* replace with ALL for all alternatives */} /> </View> ); } } AppRegistry.registerComponent(‘BarcodeApp’, () =>BarcodeApp);
Properties
- onBarcodeRead
Will call the specified method when a barcode is detected in the camera’s view. The event contains data (barcode value) and type (barcode type). The following barcode types can be recognized:
BarcodeFormat.UPC_A BarcodeFormat.UPC_E BarcodeFormat.EAN_13 BarcodeFormat.EAN_8 BarcodeFormat.RSS_14 BarcodeFormat.CODE_39 BarcodeFormat.CODE_93 BarcodeFormat.CODE_128 BarcodeFormat.ITF BarcodeFormat.CODABAR BarcodeFormat.QR_CODE BarcodeFormat.DATA_MATRIX BarcodeFormat.PDF_417
- torchMode
Values: on, off (default) Use the torchMode property to specify the camera torch mode.
- cameraType
Values: back (default), front
Use the camera Type property to specify the camera to use. If you specify the front camera, but the device has no front camera, the back camera will automatically come into use.
Conclusion
The React Native Barcode scanner offers a great facility for scanning barcodes. Indeed, in today’s increasingly commercialized environment, barcode scanning has become a must-have feature for every smartphone. Therefore, we have brought you the very positive aspects of React Native Barcode Scanner and its associated ‘know-how’.
For more information, feel free to contact our Metizsoft team. We are mobile developers and build mobile apps through the react native framework.
Read More:
React Native Firebase Integration
Complete Guide of React Native DOC Scanner Only for iPhone
React Native Image Crop Picker Android
AboutChetan Sheladiya
Related Posts
How is Android 11 Features Going to Impact Your Mobile Application?
So, it’s the talk of the industry; Android 11 is the latest upcoming OS from Google. Android 10 has already brought...
How Swift Wins Over Objective-C for iPhone Application Development?
Web & Mobile applications have completely redefined the meaning of ‘Convenience/Comfort’. The iPhone Application is...